Simple shortcuts for Smooth Imports in Node.js
- Published on
- • 2 mins read•––– views
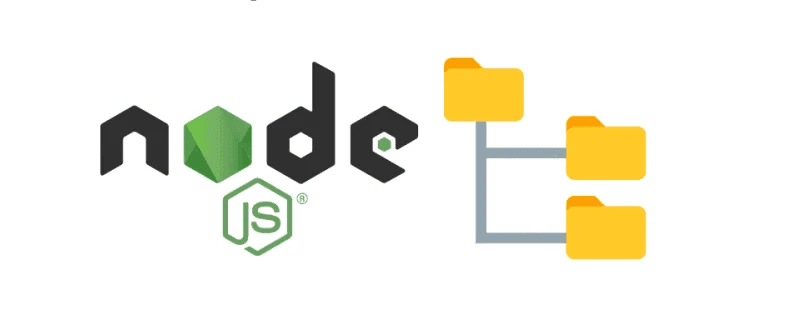
Change directories to this, and that, and then that... until you finally find the file! 😩 Doesn’t it feel like a wild goose chase when you have something like this?
import { fetchUserData } from '../../../../../services/userService'
import orderController from '../../../../../controllers/orderController'
It's like trying to navigate a maze with no map! One great solution to this "mess" is using module aliases, which let you simplify your import statements and reclaim your sanity:
import { fetchUserData } from '@services/userService'
import orderController from '@controllers/orderController'
Now, your code is cleaner, easier to read, and a whole lot more fun to work with! 🎉
In this case, @services
points to ./src/services/*
, and @controllers
points to ./src/controllers/*
.
Steps to Set Up:
Update
tsconfig.json
: Add the following configuration to specify the base URL and paths:"compilerOptions": { "baseUrl": "./src", "paths": { "@controllers/*": ["controllers/*"], "@services/*": ["services/*"] } }
Install
module-alias
: Run this command to add the package:npm install --save module-alias
Then, configure it in your
package.json
:"_moduleAliases": { "@root": ".", "@services": "dist/services", "@controllers": "dist/controllers" }
Register the Module: Import
module-alias
in your entry file (e.g.,index.ts
):require('module-alias/register')
Now you can use your new aliases throughout the project. For example:
const userService = require('@services/userService')
const orderCtrl = require('@controllers/orderController')
Or using ES6 syntax:
import userService from ('@services/userService')
import orderCtrl from ('@controllers/orderController')
Conclusion
With these changes, you’ll enhance your development experience by using cleaner, more manageable import paths. Reload your IDE and enjoy coding with your new aliases!
Cheers