Handling Python Dictionaries with Dot Notation
- Published on
- • 4 mins read•––– views
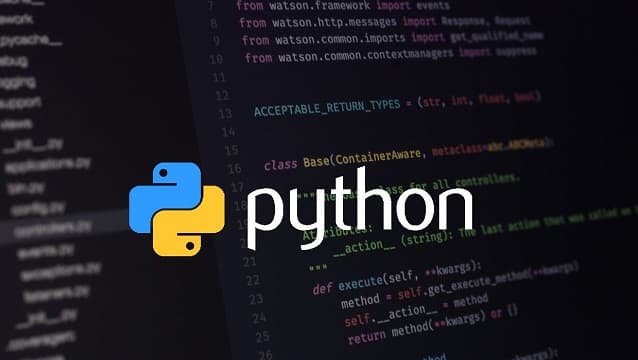
Hello folks! It's me again with some Python and JavaScript goodness. Let's explore handling Python Dictionaries with Dot Notation.😎
Case in point;
let's take this JSON file named data.json
that looks like this:
{
"user": {
"id": 42,
"name": "Alice",
"preferences": {
"language": "en",
"timezone": "UTC"
}
},
"posts": [
{ "title": "Python Basics", "date": "2023-01-01" },
{ "title": "Advanced Python", "date": "2023-01-15" }
]
}
In JavaScript, handling this data is quite straightforward using dot notation:
let userData = {}
loadUserData = async function () {
const response = await fetch('https://example.com/data.json')
if (response.status === 200) userData = await response.json()
}
displayUserName = function () {
console.log(userData.user.name)
}
loadUserData().then(displayUserName)
Output:
Alice
However, when switching to Python, trying to access attributes using dot notation directly results in an error:
import json
def main():
with open('data.json', 'r') as f:
data = json.load(f)
print(data.user.name) # Raises AttributeError
if __name__ == "__main__":
main()
To access values, you must use the conventional dictionary syntax:
print(data["user"]["name"]) # Output: Alice
Achieving Dot Notation in Python Dictionaries
Although Python doesn't provide dot notation natively for dictionaries, you can use a custom class to mimic this behavior.
Using a Custom Class
import json
class DotDict:
def __init__(self, **entries):
self.__dict__.update(entries)
def main():
with open('data.json', 'r') as f:
data = DotDict(**json.load(f))
print(data.user.name) # Output: Alice
if __name__ == "__main__":
main()
However, this method does not allow for easy access to nested attributes:
print(data.user.preferences["language"]) # Output: en
Using SimpleNamespace
A more elegant way to achieve dot notation is by using SimpleNamespace
from the types
module:
import json
from types import SimpleNamespace
def main():
with open('data.json', 'r') as f:
data = SimpleNamespace(**json.load(f))
print(data.user.name) # Output: Alice
if __name__ == "__main__":
main()
Yet again, accessing nested attributes still requires dictionary syntax:
print(data.user.preferences["timezone"]) # Output: UTC
Utilizing Community Packages for Dot Notation
Fortunately, the Python community has developed several packages that allow you to use dot notation with dictionaries. Here are two popular options:
Prodict
Installation:
pip install prodict
Usage:
import json from prodict import Prodict def main(): with open('data.json', 'r') as f: data = Prodict.from_dict(json.load(f)) print(data.user.name) # Output: Alice # Adding a new attribute data.user.age = 30 print(data.user.age) # Output: 30 if __name__ == "__main__": main()
Note: For lists of dictionaries, you will need to create a new
Prodict
instance:print(Prodict.from_dict(data.posts[0]).title) # Output: Python Basics
Limitations:
- You cannot use dict method names as attribute names because of ambiguity.
- You cannot use Prodict method names as attribute names(I will change Prodict method names with dunder names to reduce the limitation).
- You must use valid variable names as Prodict attribute names(obviously). For example, while '1' cannot be an attribute for Prodict, it is perfectly valid for a dict to have '1' as a key. You can still use prodict.set_attribute('1',123) tho.
- Requires Python 3.7+
Python-Box
Installation:
pip install python-box
Usage:
import json from box import Box def main(): with open('data.json', 'r') as f: data = Box(json.load(f)) print(data.user.name) # Output: Alice print(data.posts[1].title) # Output: Advanced Python if __name__ == "__main__": main()
Note: Python-Box effectively handles nested dictionaries within lists without requiring additional instances.
Conclusion
In summary, while Python does not support dot notation for dictionaries natively, you can achieve this functionality through community packages such as Prodict and Python-Box. Personally, I find Prodict to be particularly suitable due to its lightweight nature and flexibility in handling undefined keys, akin to JavaScript:
if (userData.user.age === undefined) { // JavaScript code }
if data.user.age is None: # Python code
# Handle non-existing key
Happy coding!