How to Manage Python Dependencies and Environments with Poetry
- Published on
- • 3 mins read•––– views
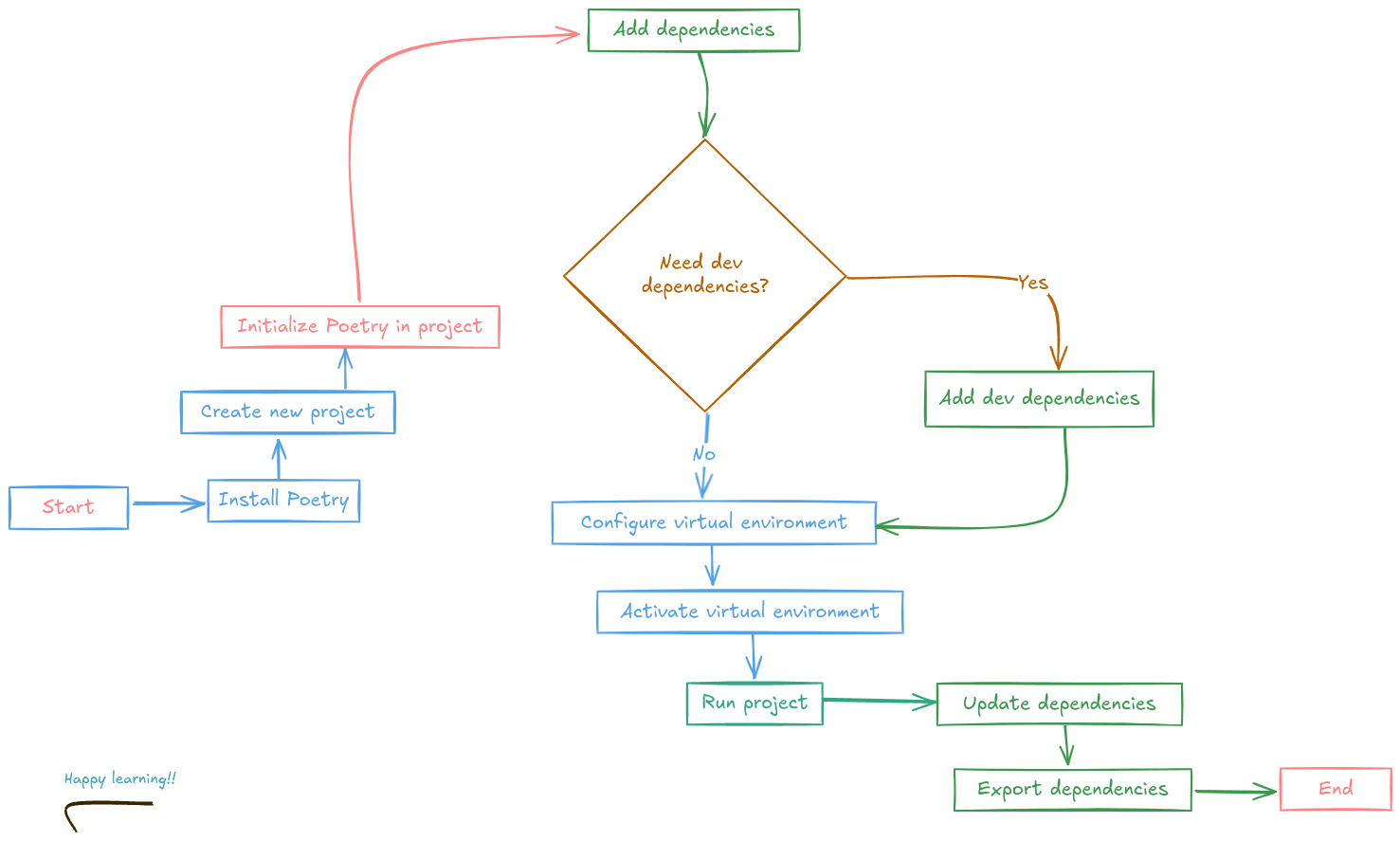
Did you know that setting up a Python project locally can quickly become a crisis due to different operating systems, Python versions, and poor dependency management? I can't imagine that hassle, especially in large projects.
But here's a personal favourite Solution: Use Poetry, a modern tool for dependency management and packaging in Python.
Python 3.7+ Required
Step 1: Install Poetry Globally
Install Poetry with:
curl -sSL https://install.python-poetry.org | python3 -
Step 2: Initialize a New Project
Create a new project:
poetry new demo_project
cd demo_project
This creates a directory structure like:
.
├── demo_project
│ └── __init__.py
├── pyproject.toml
├── README.md
└── tests
└── __init__.py
Step 3: Add Dependencies
To add dependencies, such as requests
and flask
:
poetry add requests flask
Your pyproject.toml
will update to include:
[tool.poetry]
name = "demo-project"
version = "0.1.0"
description = "Demonstrating how to manage Python dependencies and environments with Poetry."
authors = ["Joseph"]
readme = "README.md"
[tool.poetry.dependencies]
python = "^3.11"
requests = "^2.32.3"
flask = "^3.0.3"
[build-system]
requires = ["poetry-core"]
build-backend = "poetry.core.masonry.api"
Step 4: Install All Dependencies
Install all dependencies specified in your pyproject.toml
:
poetry install
Step 5: Activate the Virtual Environment
Activate your project’s virtual environment:
poetry shell
Step 6: Remove a Dependency
To remove a dependency like flask
:
poetry remove flask
This updates your pyproject.toml
and poetry.lock
files automatically.
Step 7: Let's set-up a simple flask app
In the demo_project
folder, create a main.py
file and paste in the following:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def home():
return "Home page, yes?"
def main():
app.run(debug=True)
if __name__ == '__main__':
main()
Your folder structure should now look like so:
.
├── demo_project
│ ├── __init__.py
│ └── main.py
├── pyproject.toml
├── README.md
└── tests
└── __init__.py
Step 8: Define and Run a Script
Add a script to your pyproject.toml
under [tool.poetry.scripts]
:
[tool.poetry.scripts]
app = 'demo_project.main:main'
As such, your configuration will look something like this:
[tool.poetry]
name = "demo-project"
version = "0.1.0"
description = "Demonstrating how to manage Python dependencies and environments with Poetry."
authors = ["Joseph"]
readme = "README.md"
[tool.poetry.dependencies]
python = "^3.11"
requests = "^2.32.3"
flask = "^3.0.3"
[build-system]
requires = ["poetry-core"]
build-backend = "poetry.core.masonry.api"
[tool.poetry.scripts]
app = 'demo_project.main:main'
Run your application with:
poetry run app
Conclusion
These examples demonstrate how Poetry simplifies Python project management, particularly with dependency handling. I hope you found this blog helpful!
Thank you for reading and happy Coding!